The wobble is not the obstacle, it's the way
| life, parenting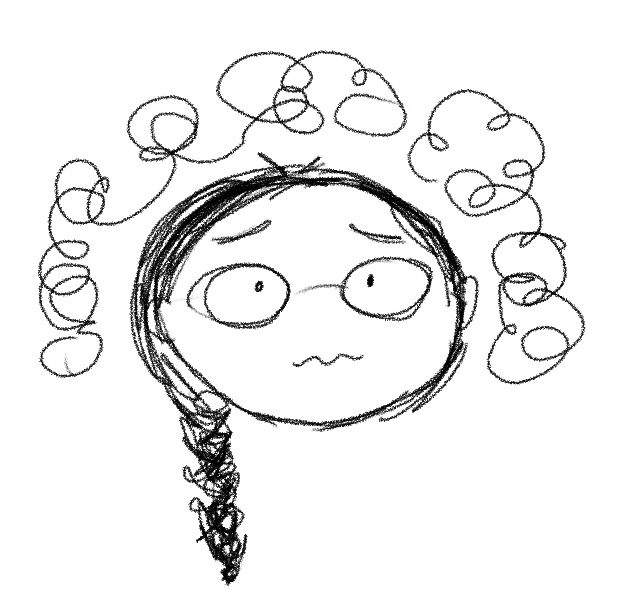
9 PM on a schoolday, time for me to nudge A+ off to bed. A+ is clicking through the Stardew Valley wiki, slurping up all sorts of trivia that she'll probably trickle into our conversations. There are two pieces of homework left to do, one with quite a few slides to complete. And drawing. She's not a big fan of drawing assignments. "My hand is tired," she says.
I try to be calm and supportive. I wobble. Could've done this earlier, I think. I manage to keep myself from saying it. I teeter, noticing myself mentally fast-forwarding decades ahead. Oh no, she's not going to get the hang of doing things that she finds boring, she won't develop study skills or executive control, she'll cram through all the classes she can coast in, and all of it will come crashing down in university when she might actually need to buckle down and study.
She's 9! She's a long way from university.
I'm learning to embrace my anxiety and appreciate how it tries to keep us all safe. This feeling makes sense. I want to help her avoid mistakes, especially when the feedback cycle is long and the results of choices will only be seen much later.
But anxiety gets in the way of parenting. If I let the fearful part of my brain take over, I'll inadvertently teach her that mistakes are catastrophic rather than just ordinary Tuesdays. I want to hold her steady, but the wobbles are how we learn.
It's somewhat manageable now, when we can talk about these things openly. A+ can laugh off my worries ("Mom, you're fretting again,") and W- can remind me to slow down when it runs away with me. He's usually pretty chill about all this. It'll be harder when the cognitive rewiring of puberty or menopause turn ordinary conversations into minefields right when the stakes get higher. The more I tighten my grip, the more star systems will slip through my fingers. (There I go again with catastrophizing.)
Besides, I want to help A+ avoid the paralysis of perfectionism or self-recrimination. I want her to be able to experiment, and to pick herself up and try again if things don't work out the first time around. To do that, I need to learn to change my perspective from being anxious about mistakes to seeing the opportunities for re-takes.
There are many things I can't teach A+. Some things can't fully be taught, they can only be learned, like how to balance the clay on the pottery wheel. Sometimes I don't even know what the right answer would be, like what kinds of tips work for her particular brain. Some things change over time and she'll need to change with them, like how to adapt to life's situations. She'll need to learn how to learn instead of relying on one fixed answer.
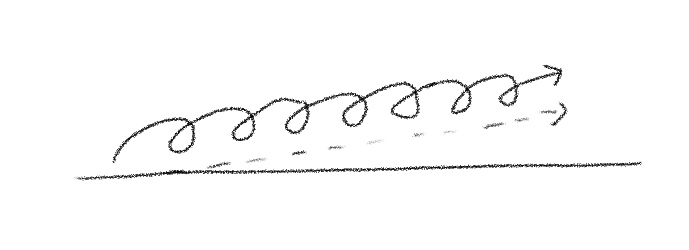
Fortunately, life comes with so many opportunities to practise. The Toronto public school calendar has 187 instructional days, so she gets plenty of chances to manage her homework and get feedback. The repetitive nature of things used to frustrate me when it came to my tasks (always more dishes to wash, always more clothes to fold), but it's good for learning, especially while the stakes are low. It's her experiment, I remind myself. About half the time, she doesn't even want my help. ("I can do it, Mom.") She's sensible enough to try things out on small experiments instead of scary ones: shopping at the grocery store on her own, not skydiving.
There's plenty of stuff for me to learn while she learns. When I get the urge to correct her work ("How does that line up with the rubric?") or nag her to get her work done, I tell myself:
- Is it really a problem? The teacher isn't expecting her to completely master all the skills, and the teacher is in a good place to give developmentally-appropriate feedback. I can let her experiment with how much work she wants to put into things, and she can see what that results in. Despite all my twitchiness about how she puts off her daily homework until 9 PM, she still manages to get things done. Judging from the frequent reminders her teacher gives the virtual class, she's probably ahead of the curve. So maybe it's not a problem.
- Whose problem is it? Something might not be my problem. It might not even be her problem. She reads during class time, for instance. Sometimes she misses something that can't be figured out from just the homework slide deck. Maybe that's partly her experimenting to find the right balance between attention and stimulation. Maybe that's also partly a consequence of how school is designed to go at the group's pace. Not entirely her problem.
The more I let go of the small stuff, the more experience she'll be able to draw on for the big stuff. I hope she'll get the hang of thinking of life as mostly series of little experiments, and to notice when there's a bigger choice that needs more thinking because it's more long-term. The more she decides, the more confidence we both develop in her decisions.
This reminds me of how kids learn how to bike. The popular approach uses training wheels to prevent falls. The idea is to gradually raise them as the kid improves, but I usually see kids pedaling along (perhaps slightly leaning over to one side) to match the slowness of parents' walking. It's hard to balance when you're going slow. But pedaling isn't the hard part. Balancing is, and you develop balance by balancing. Maybe that's a little like how I get tempted to rescue A+ from the results of some of her choices, but letting her try things is how to help her learn.
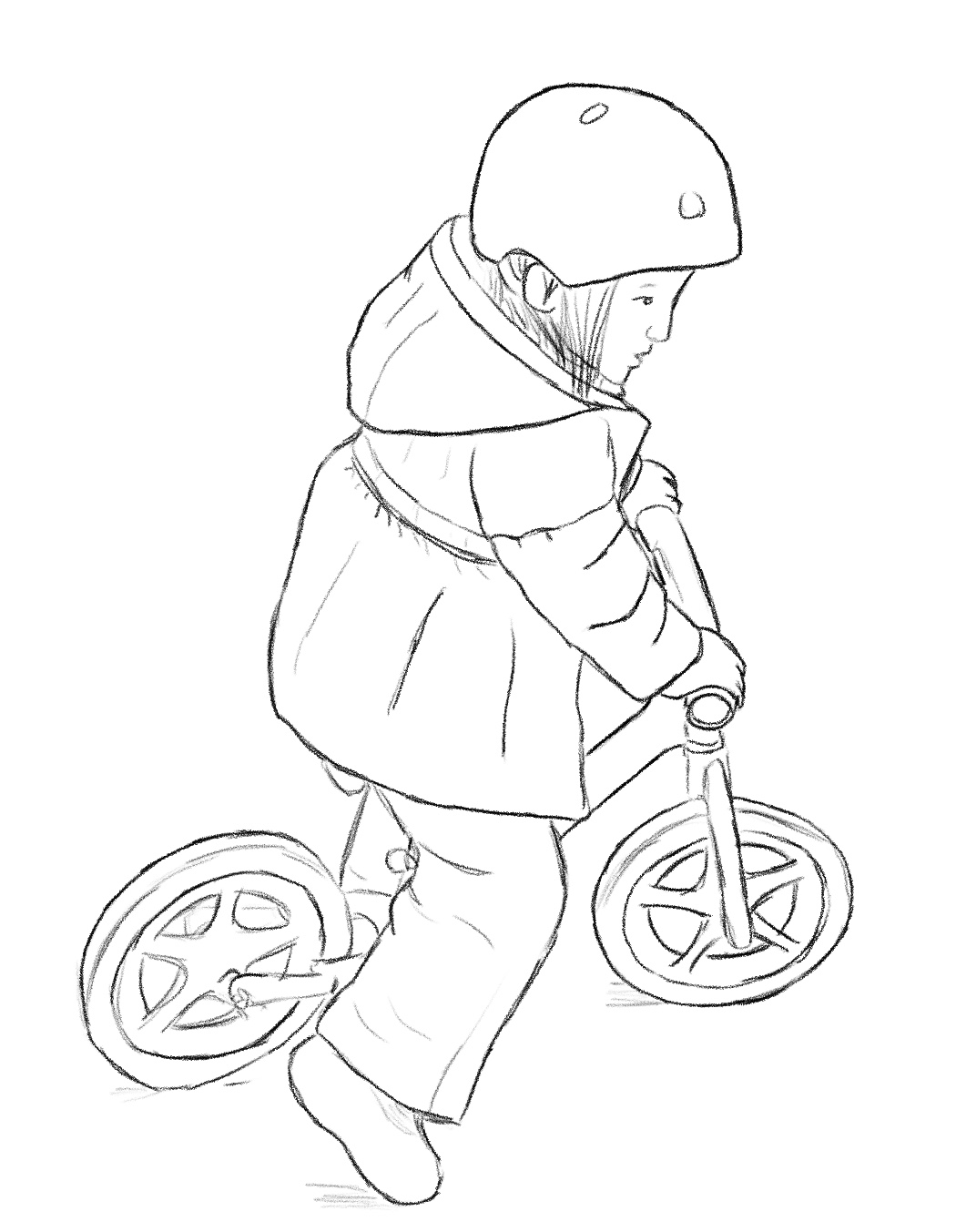
A+ learned how to bike using a balance bike instead of using training wheels. When she was two, she toddled along on a Strider, which was light enough for her to manage. Eventually she figured out coasting. She was proud of being able to do it on her own. Then we upgraded her to a Cleary Gecko freewheel bike, with proper hand-brakes and everything. After a few attempts with us holding her under her armpits, she was ready for us to steady her with a hand on her back, and then for us to be close, and then she was off on her own. She fell and skinned her knee many times, developing an appreciation of pants for protection and ice cream for comfort. The more she biked, the more she learned how to notice that feeling of being slightly off-balance, and the better she got at correcting it. Now we can bike on the streets together.
You can't learn how to bike if the training wheels are always on, or if someone's always holding you steady. It's okay to wobble and fall and get up. You learn that you can survive a skinned knee, and so you keep going.
Sometimes, when A+'s in the middle of a meltdown, I have to remind myself not to try to fix it in the moment. That doesn't work, anyway. Just take the loss and try again next time. Sometimes, once we've both calmed down, I ask A+ to imagine rewinding back to a situation so we can play it out a little differently. Sorry, I meant to say this, not that. Would that work better? Next time.
Not mistakes. Data. Just another step in the journey.
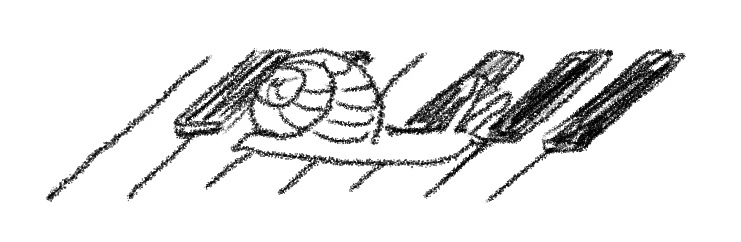
Getting better at getting better helps me, too. I've been practising piano, making steady progress through the Simply Piano app. I've been playing for about four months now. I took piano lessons as a kid, but not to any serious extent. Back then, I got bored with the simple exercises I had to do. Now I feel slow, snail-slow, but I can savour the way my mind is beginning to get the hang of things, knowing that it will take me many tries to get the hang of it. I'm starting to be able to look at the notes and remember the phrases, imagine what the next sequence will sound like before I play it, and notice how my hands move to make it happen.
When my fingers wobble on the keys, I slow down and try again. There's no point in berating myself. If my mind keeps hiccuping or my fingers keep stumbling, I can think: ah, is this because I'm tired, or because I want to do something else, or just because I'm learning and it takes time to get the hang of things? I'm getting better at figuring out when I should probably call it a day so that I don't practise mistakes into my muscle memory and when I might benefit from just slowing down the segment.
I still stumble through pieces I've successfully played before. Remembering is hard. But I'm getting better at being patient with myself, accepting that it's because I'm still in the middle of the journey. It's not a mistake that I should grump at myself about. It's just part of a re-take. This is what learning looks (and sounds) like. Of course it doesn't start out perfectly smooth.
Here's me learning Mozart's "Rondo alla Turca", with the app providing accompaniment in the background. It's not perfect, but it's progress.
We were at the playground. I ate the remaining crackers in the snack box because I thought A+ was done. Turns out she was saving them for later. She was very upset. I apologized and promised to ask next time, but she was too far gone to hear.
That was a tough moment. A+ was already emotionally off-balance because the playdate hadn't gone as well as it usually does. Discovering I had eaten the crackers she was looking forward to was the last straw. She dissolved into tears. I snuggled her and settled in for a long wait. I think: Where's the line between comforting her and coddling her? Does my anxiety teach her this is too hard to handle? We're not quite at the point of being able to shrug off mistakes. I remind myself that she'll learn what she's ready to learn.
Looking around while A+ drenched my left shoulder, I noticed a skateboarder on the park road. Maybe a man in his thirties? He was trying to jump his skateboard over a low concrete lane divider. He had been at it for a while before I noticed. Most times, he was able to clear the divider, but the skateboard slowed down too much on the other side and he had to jump off. On the seventh try that I saw, he landed back on the skateboard and rolled on for a bit. Success! He tried again and failed. Four more failures before his next success. One more attempt–another failure–and then he called it a day. I'm sure he'll be back at it.
A+ continued to cry. My phone buzzed, reminding me that we probably wanted to get going before the rain in the forecast. I carried her as I picked up our bags and put them in my bike. Eventually I needed to gradually ease her off me. She curled up in the bucket of my front-loader cargo bike, still crying. I tucked the towel around her like a blanket, buckled her bike into the tow-bag, and walked the bikes home. She fell asleep.
A wobble, a fall. But I'm sure we'll be back at it too. (And we did; the next day, she was happily playing with her friends again.)
It's hard to be in the moment. Sometimes the moment sucks. It's hard to be far ahead in the future. It makes decisions feel too big. Do-overs make things just the right size. If we can get good at shrugging off the inevitable failures and treating them as data so that we can sketch out ideas for the next experiment, I think that'd be pretty cool. Instead of "Oh no!" or even "Are you sure about that?" (what kid likes to be doubted?), I can lean towards, "Hmm, let's find out."
As predicted, we had another late-night homework situation. This time she had a headache and wanted to go to bed, homework unfinished. I was able to let go and just focus on snuggling her in. The next day, after morning routines and without any nagging, she did the homework and submitted it. Late, but done.
There'll be another bedtime homework session, I'm sure. I have to trust that even though I want to shortcut the learning for her, she's got this. She's figuring things out. If we stumble, that just helps us practice for next time, and there are so many opportunities to try again. The wobble is not the obstacle, it's the way.1
This post is yet another take on the June IndieWeb Carnival theme of Take Two. Here are two other ones: Making and re-making: fabric is tuition and Thinking about time travel with the Emacs text editor, Org Mode, and backups.
Related reflections:
Footnotes
Related: The Obstacle is the Way, Ryan Holiday's book on Stoicism; the title rephrases this thought from Marcus Aurelius's Meditations: "… and that which is an obstacle on the road helps us on this road."